Cacatoo overview
Next tutorial →
In this tutorial, I will briefly explain:
- Why I made Cacatoo
- Getting started with Cacatoo
- The basic structure of a Cacatoo model
- The advantage of a dynamic programming language
Why I made Cacatoo
Why grid-based models?
When implementing individual-based models, there are a number of important decisions to make. When the primary interest is spatial structure, the most important decision is whether space is continuous or discrete. While modelling individuals in continuous space is very powerful when modeling flocking behaviour (Papadopoulou et al, 2021), foraging strategies (van der Post & Semmann, 2011), or biofilm mechanics (Kreft et al., 2001), it is often computationally infeasible to model increasingly large populations. Thus, when one is for example interested in evolution, grid-based models are a much more powerful tool to simulate a continuous process of mutation and selection. Although these grid-based simulations may appear somewhat "unrealistic", it is important to note that realism is not what necessarily defines a good model. Instead, A is a good model of system B, when studying A teaching you something about B (Paulien Hogeweg, 2011 lecture on Computational Biology). I have published numerous papers that were based primarily on grid-based models, and I can personally testify to having learned a lot through grid-based modelling, no matter how weird and "pixely" they may look!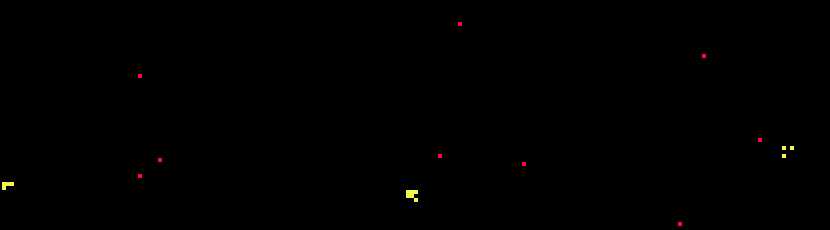
A more user-friendly CASH
When working with individual-based models, direct visual feedback is important. Not only are you more likely to detect programming mistakes, but it also aids rapid exploration of parameter space. Over the last decade, most of my models were implemented by using the CASH C-library, which would allow direct visual feedback by means of the X11 library. CASH was originally developed by R.J. de Boer & A.D. Staritsk, and was then further developed by Nobuto Takeuchi (CASH2s) and myself (CASH2.1). However, as it is based in C, developing models with CASH requires a lot of programming experience, and even an experienced user like myself can sometimes take days to track down a simple bug. Moreover, sharing your model with other users can be a pain in the neck, as installation is slightly different depending on the operating system. For this reason, I have been trying to find a new programming language in which to implement something that is reminiscent of CASH. Even though some of the new programming languages (e.g. Ruby, Julia) are very fast, creating a display for direct visual feedback typically slowed things down tremendously.The new and improved Javascript
After searching for a new programming language for CASH for years, Inge Wortel and Johannes Textor published Artistoo, a toolbox to simulate cell tissues with 100% Javascript (see animation below). They even compared this toolbox with other known C++-libraries, and show their toolbox is not significantly slower. This initially surprised me, as I remember Javascript being a language that was mostly use to make websites more annoying, and I did not expect it to be fast. As it turns out, it wasn't always this fast. Google has invested a lot of money in optimising Javascript, especially for their own V8-engine in the Chrome browser. After fiddling around with Javascript a bit myself, I realised this was exactly what I needed to make a new-and-improved version of CASH. And because silly acronyms are an essential component of being a good biologist, the tool is now called Cacatoo: Cash-like cellular automaton toolkit (full credits for this to Jeroen Meijer).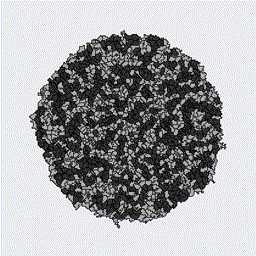
Simple cell-sorting modelled in Artistoo
Getting started with Cacatoo
Explore Cacatoo online (JSFiddle)
The easiest way to get started with Cacatoo is to go check out one of the JSFiddle examples. JSFiddle is an online, free-to-use coding platform. It can be used without an account (although making account has some benefits). You can simply click the "Fork" button, and start playing with Cacatoo. No need to download or install anything!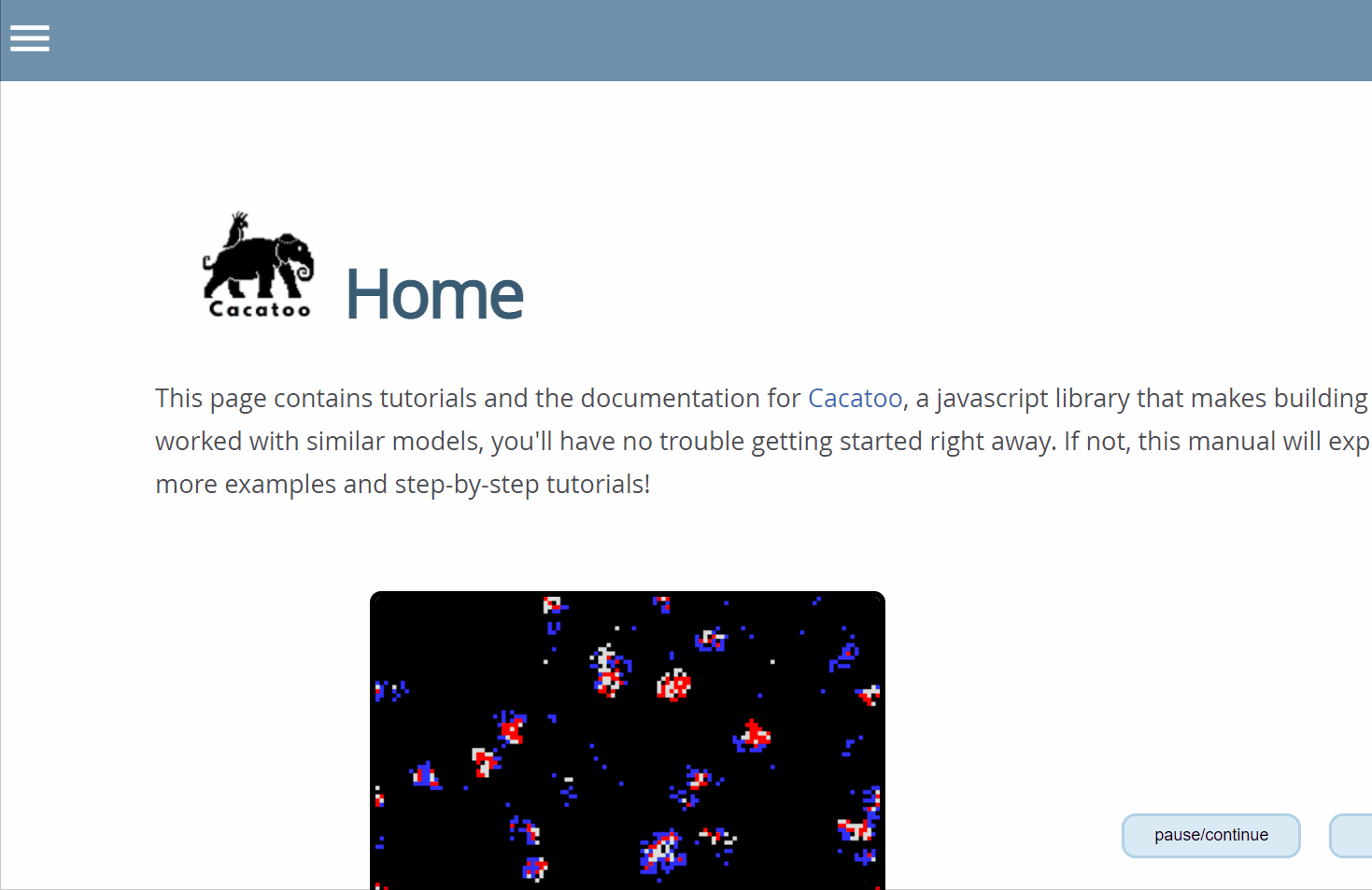
Get it on Github
If you're more interested in developing, you may want to actually get your own copy of the code. If so, then the best way to get your hands on Cacatoo is to go to the Github page and download the code (either clone it using 'git clone', or click 'Code', and then download the zip) directly from Github. Then, you can simply start checking out (and modifying!) the files in the examples directory.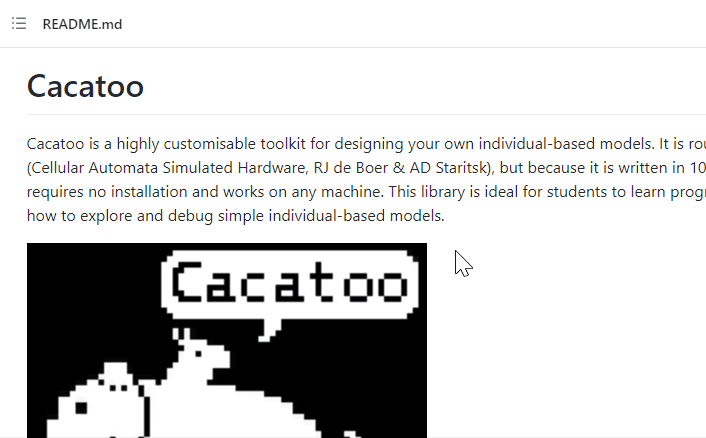
The basic structure of a Cacatoo model
1. Setup
In the setup, one defines the basic parameters of the model, including a name, the width/height of the grid, the duration of the simulation, etc. Once that is done, it is time to populate the grid with individuals (or other types of entities) and make sure these are displayed however you want them to be displayed.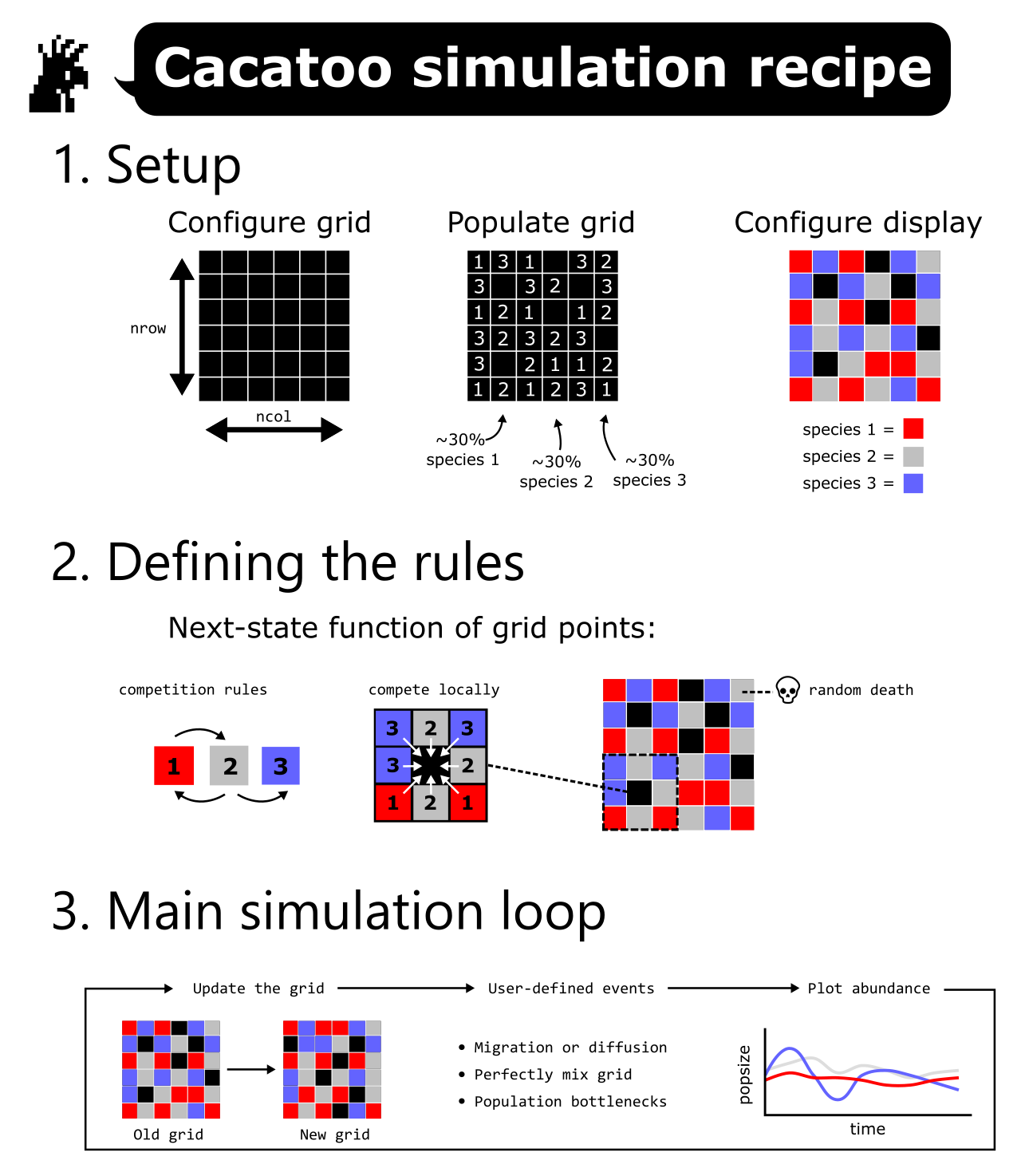
2. Defining the rules
In step 2, one defines the actual rules of each grid point. For example, you can allows species to compete for empty spots, individuals may die stochastically (or through some deterministic process!), etc. Go nuts!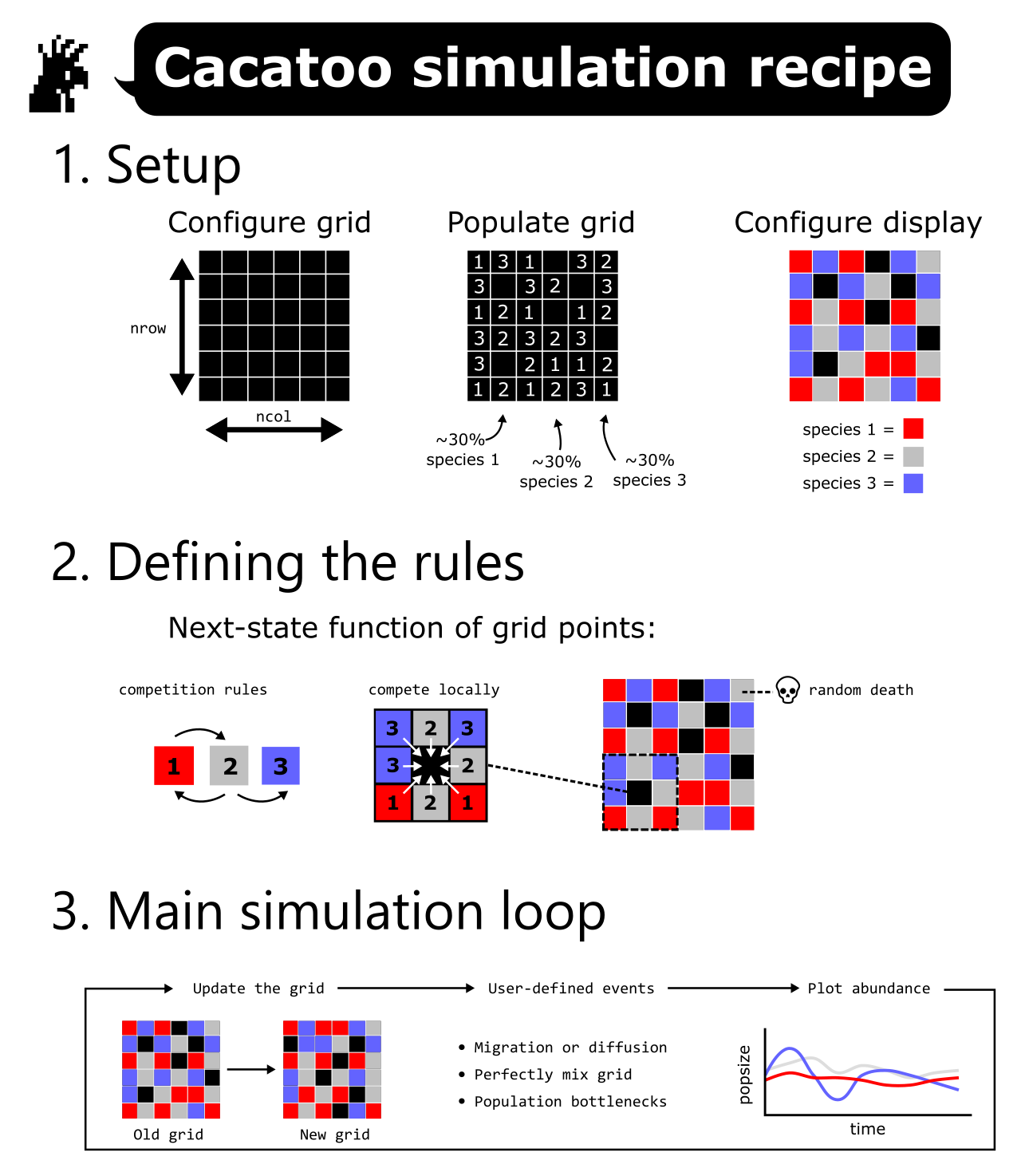
3. Main simulation loop
Finally, one defines what the main simulation loop is. This includes the updating of the grid (i.e. applying the rules defined in step 2 to the whole grid), mixing the grid, occasional bottlenecks, etc. Here, you can also add plots to visualise the results of your simulation in real time!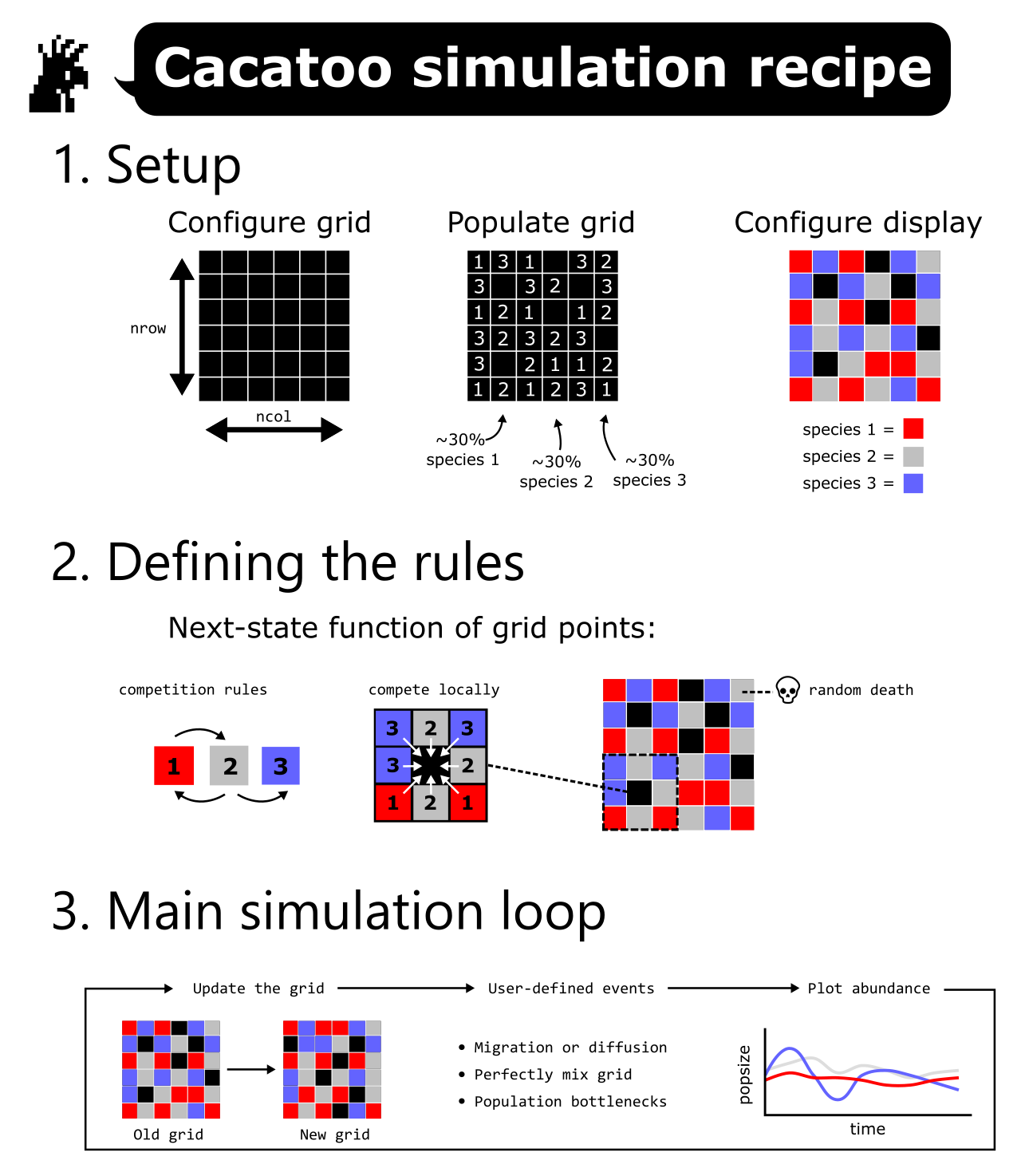
Example code
The code below illustrates what steps 1 through 3 will look like for a simple Cacatoo model. It's not that many lines of code! // NOTE: For a full version of this code with explanatory comments, see 01_GoL.html in the examples directory.
// 1. SETUP. First, set up a configuration-object. Here we define how large the grid is, how long will it run, what colours will the critters be, etc.
let config = {
title: "Cacatoo example",
description: "My first Cacatoo model",
maxtime: 50000,
ncol : 200,
nrow : 200,
scale : 2,
statecolours: {'alive':{1:'white'}},
}
// 1. SETUP. (continued) Now, let's use that configuration-object to generate a new Cacatoo simulation
sim = new Simulation(config)
sim.makeGridmodel("gol")
sim.initialGrid(sim.gol,'alive',1,0.5)
sim.createDisplay("gol","alive","Game of life (white=alive)")
// 2. DEFINING THE RULES. Below, the user defines the nextState function. This function will be applied for each grid point when we will update the grid later.
sim.gol.nextState = function(i,j)
{
let neighbours = sim.gol.countMoore8(this,i,j,1,'alive')
let state = this.grid[i][j].alive;
if(state == 0 && neighbours == 3)
this.grid[i][j].alive = 1;
else if(state == 1 && (neighbours < 2 || neighbours > 3))
this.grid[i][j].alive = 0;
else
this.grid[i][j].alive = state;
}
// 3. MAIN SIMULATION LOOP. Finally, we need to set the update-function, which is the mainwill be applied to the whole grid each time step.
sim.gol.update = function()
{
this.synchronous()
}
sim.start()
The advantage of a dynamic programming language
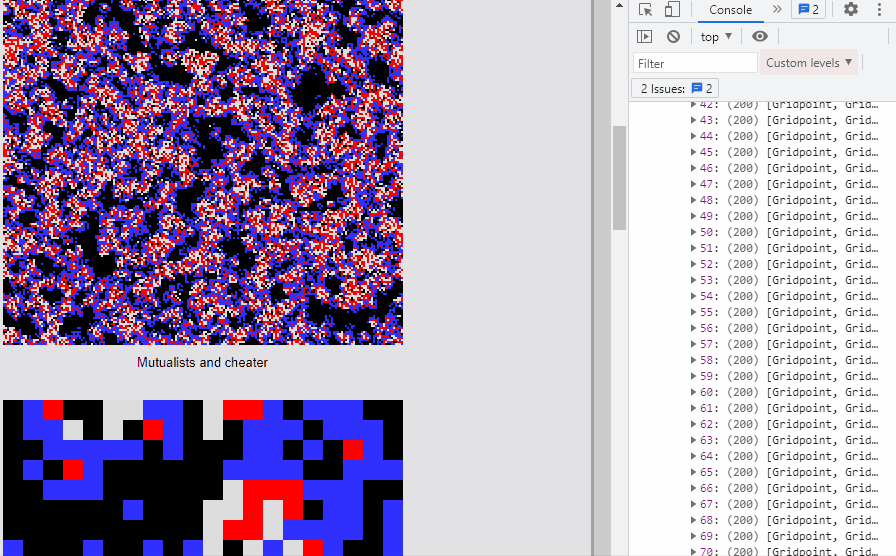